I have made JavaScript a major part of my development career for a decade now (since the >shudder< IE6 days!) so I have had my fair share of JavaScript interviews. If I have not been in an interview for even a few months I try to cram the important topics that do not come up at work too often. The simplest way to do that is review all the parts of:
JavaScript: The Good Parts by Douglas Crockford
that I highlighted and marked the crap out of years ago.
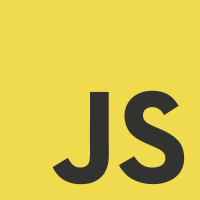
Since Douglas Crockford has spent roughly two decades explaining JavaScript concepts, it’s no surprise he simply says it better than most so the longer quotations are simply direct quotes from his book.
First quick concepts worth knowing but are easily forgotten . .
Data Types
- Six data types that are primitives (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Data_structures)
- Boolean
- Null
- Undefined
- Number
- String
- Symbol (new in ECMAScript 6)
- So there is ONE number type
- But NaN . . is also a number
- Note: isNan() is available
- For Strings and arrays
- .length (note it’s not a function but a property)
- Said best here:
- Call invokes the function and allows you to pass in arguments one by one (listed explicitly).
- Apply invokes the function and allows you to pass in arguments as an array.
- Bind returns a new function, allowing you to pass in a this array and any number of arguments.
- And this might be the best stackoverflow answer ever:
- The difference is that apply lets you invoke the function with arguments as an array; call requires the parameters be listed explicitly. A useful mnemonic is "A for array and C for comma."
- Pseudo syntax:
- theFunction.apply(valueForThis, arrayOfArgs)
- theFunction.call(valueForThis, arg1, arg2, ...)
- By putting "use strict"; at the top of your code, the interpreter will throw more errors for bad practices that are technically permitted. The major effect is it forces you to declare your variables properly and avoid using variable names that will someday be keywords.
Conditionals
- What is the difference between == and ===?
- === means “equality without type coercion” but == means that the second value will be converted to the data type of the first so that “equivalency” can be determined.
- True vs False
- Usually the concept of true and false is referred to as falsy vs truthy because the list of data types you might put in a conditional are a bit sketchy and not exactly in line with math books
- This is explained well here but in short:
- falsy: In javascript 6 things are falsy and they are- false, null, undefined, '', 0, NaN
- truthy: There are only two truthy things- true and everything that is not false
- all_things_code points out:
null == true; //false
null == false; //false!!
Objects
- ALL objects are linked to a prototype object. If you didn’t specify which after creating it, its prototype is pointing to Object.prototype
- When attempting to find a property in an object, the prototype object will be searched if the object in question is missing said value through a process called delegation.
- You can for-loop your way through the properties (not methods) of an object[1]:
for (name in objectInstance) {
//use name
}
Functions
- “A function encloses a set of statements. Functions are the fundamental modular unit of JavaScript.”
- “Function objects are linked to Function.prototype (which itself is linked to Object.prototype)."
- “The Function object created by a function literal contains a link to the outer context. This is called closure. This is the source of enormous power.”
this
- Is established at invocation of a function
- The value is determined by the invocation pattern used
- The method invocation
- When a function is a member of an object
- this is bound to that object
- The function invocation
- When a function is NOT a member of an object
- this is bound to global object (see also this)
- The construction invocation
- When a function is invoked with the new prefix . . this is bound to that new object.
- The apply invocation
- apply simply let’s you control what this is.
- Return
- If the function was invoked with the new prefix and the return value is not an object, then this (the new object) is returned instead
- Modules
- A module is a function or object that presents an interface but hides its state and implementation
- See also the “Immediately Invoked Function Expressions (IIFE)”
- Summarized best here
- “The primary reason to use an IIFE is to obtain data privacy. Because JavaScript's var scopes variables to their containing function, any variables declared within the IIFE cannot be accessed by the outside world.”
- See also:
- https://en.wikipedia.org/wiki/Immediately-invoked_function_expression
- https://www.safaribooksonline.com/library/view/javascript-cookbook/9781449390211/ch06s11.html
- Cascading
- Using a paradigm where some functions do not return values but instead change some kind of state and then return this, we can chain events and enable cascading:
- Jquery applications use this a lot!
- $('#bobsDiv').on('mouseup').styleChange('background-color', 'grey')
Arrays
- Note typeof(anArray) returns “object” so often you will also want to use Array.isArray(arrayInstance) or <Array KeyWord>.isArray(<array variable>)[2]
- .sort() sorts arrays of numbers improperly:
- It might return [ 12, 15, 2, 5]
- So you have to give it a comparison function:
arrayOfNums.sort(function(a, b) {
return a-b;
});
- this works bc it needs to return negative num if a should come first, 0 if equal and positive if the two need to be reversed
- What is the difference between foreach and map?
- foreach manipulates each member a given list but does not return anything
- map returns a new list with updated members
Based on my experience this covers a whole lot that will come up in a senior JavaScript interview. Am I missing anything major?
Much thanks to all_things_code for his input and especially his vital correction!
Much thanks to all_things_code for his input and especially his vital correction!
[1] Note different syntax from Python
[2] G3E9 points out that the Array.isArray is not supported by all browsers so for your IE8 or below users (or FF 3 users, if they exist?) so please note this simple polyfill:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/isArray
[2] G3E9 points out that the Array.isArray is not supported by all browsers so for your IE8 or below users (or FF 3 users, if they exist?) so please note this simple polyfill:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/isArray
if (!Array.isArray) {
Array.isArray = function(arg) {
return Object.prototype.toString.call(arg) === '[object Array]';
};
}